Introduction
In this blog, we will see how to write a custom trace logs and exception in Application Insights using Azure Function/Console App(Web Job).
If you are looking for a way to configure Application Insights for Azure Function then please refer below link.
https://docs.microsoft.com/en-us/azure/azure-functions/functions-monitoring
Problem Statement
We have a requirement, In which Azure function will connect to the third-party application after a specific time interval and retrieved the failed jobs. We want to logs those exceptions in Application Insights.
Prerequisites
- Access to Azure account – Admin or Co-Admin role.
- Visual Studio 2017 or above.
Note: Application Insights will capture the logs for the Apps(Any Custom C# Code) running in Azure Environment. It will not capture the logs from the application running on your local machine.
The Same code is applicable for console app running in Azure Environment as a Web Job.
Modules
- Provision Application Insights
- Create a Azure Function in Visual Studio
- Provision a Azure Function in Azure Portal
- Publish the App from Visual Studio
- Execute the Azure Function from Postman.
- Verify the Logs using Logs(Analytics)
Let’s focus on the first part and provision the Azure service.
1. Provision Application Insights
Steps :
- Login to the Azure Portal and create a new resource group.If you have existing resource group please skip this step.
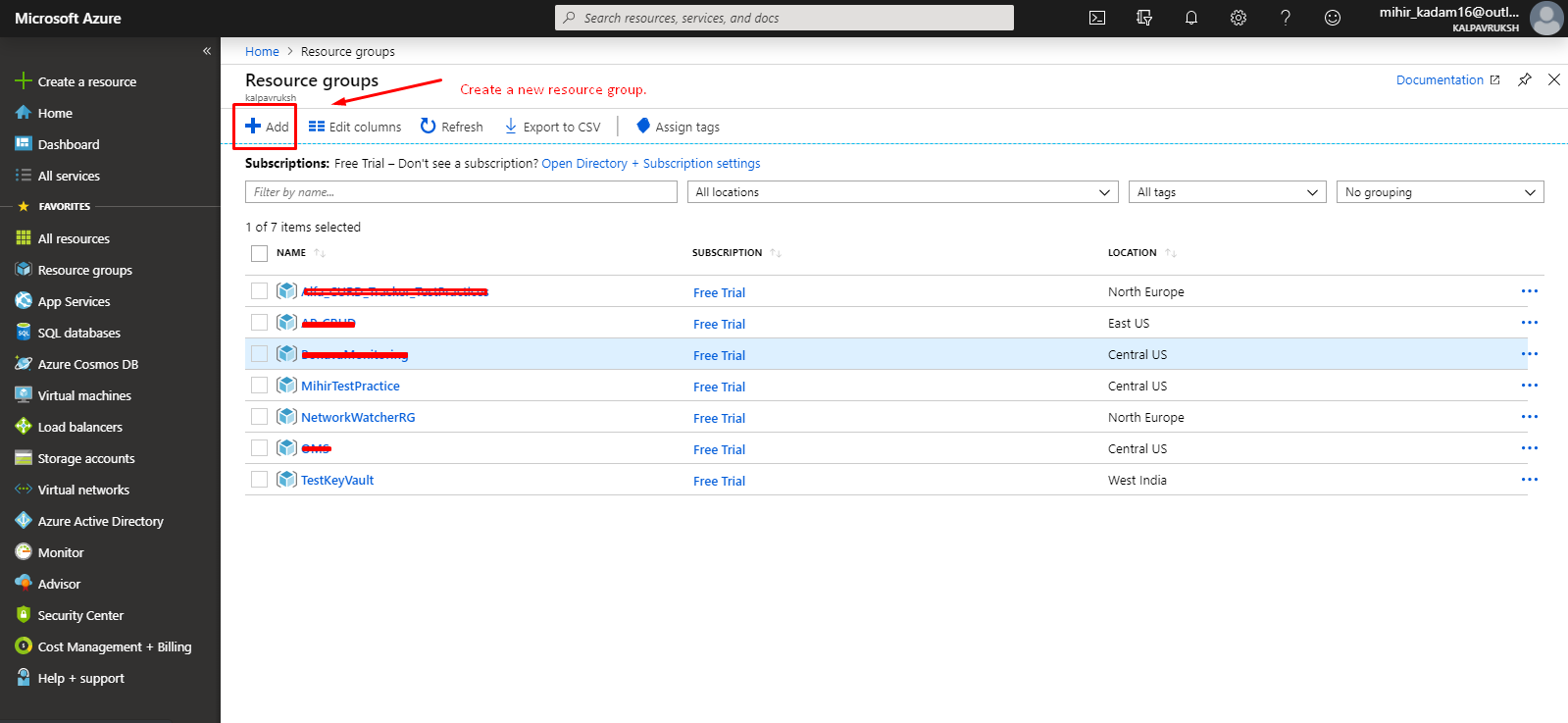

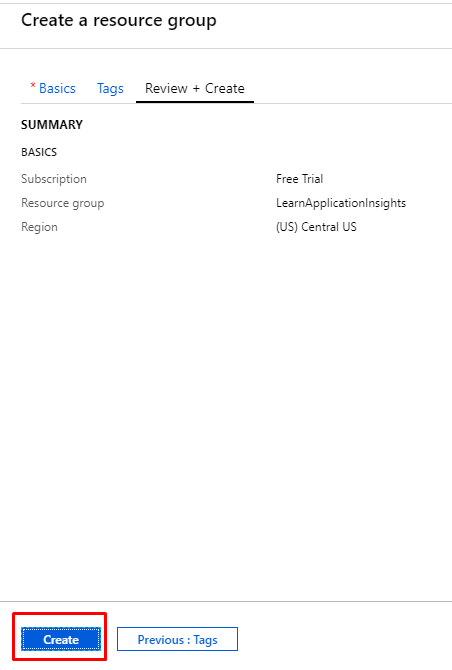
2. Open the resource group and click on + Add.
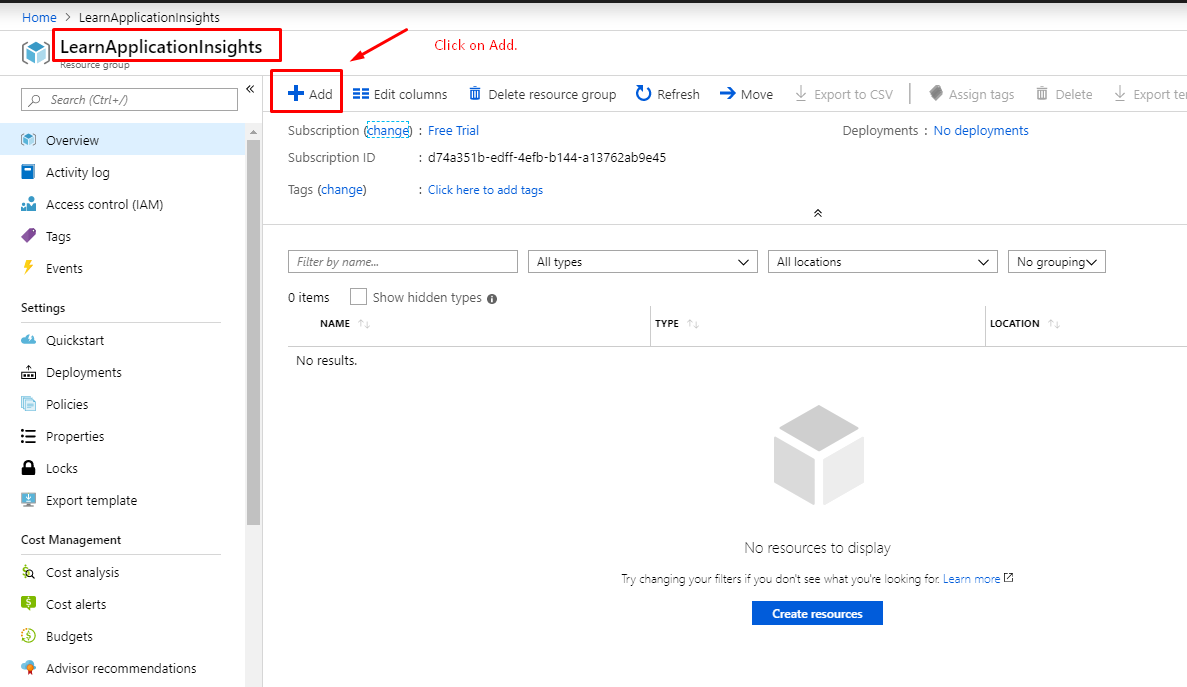
Search for Application Insights and Select the resource.
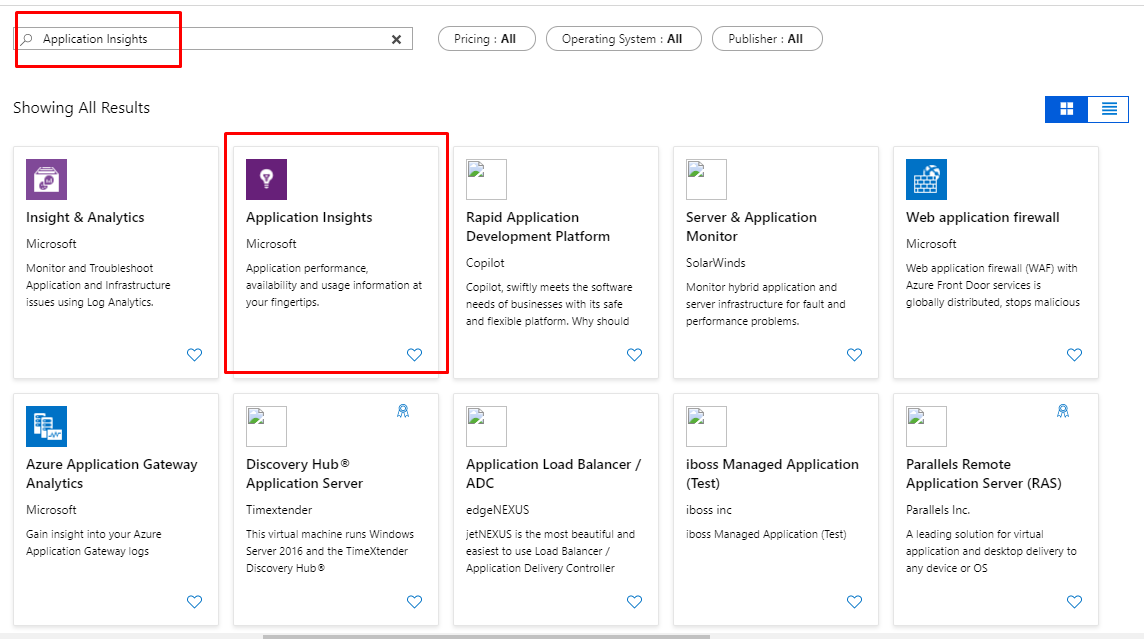

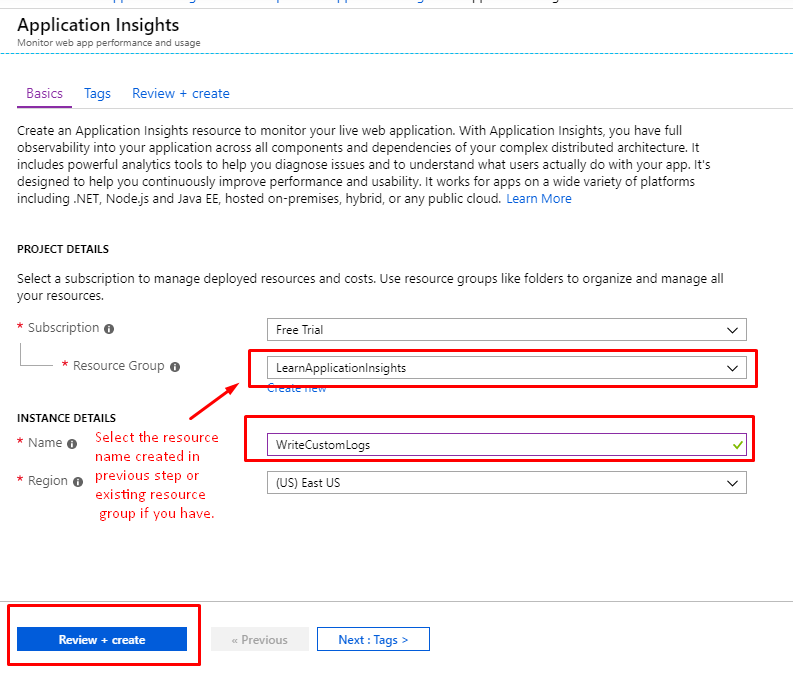
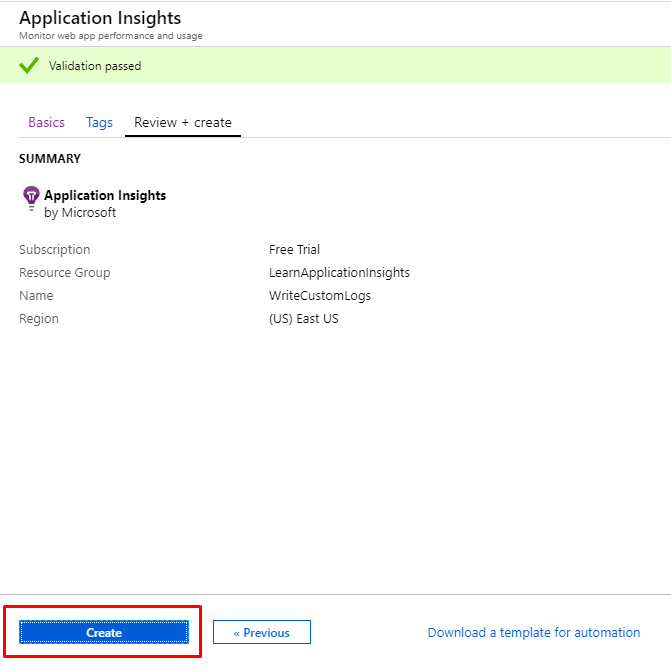
Kindly wait for the deployment process.
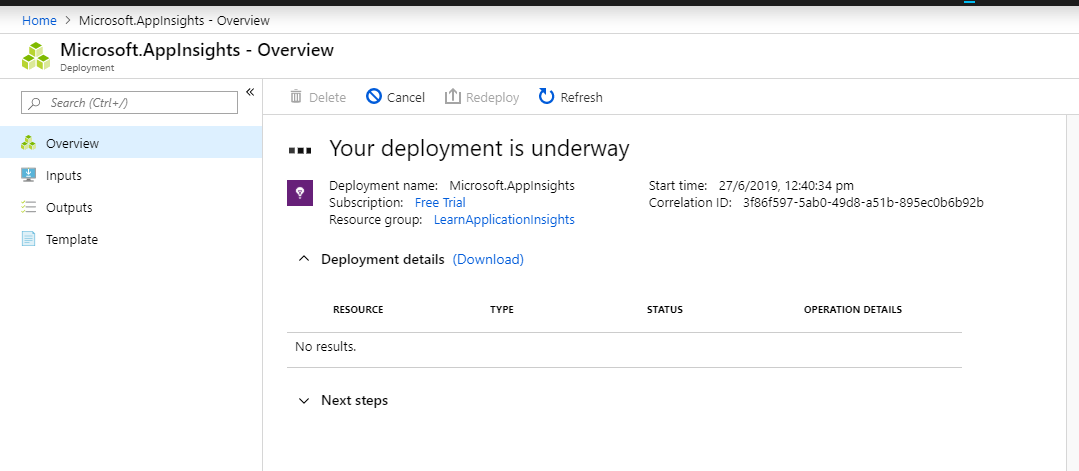
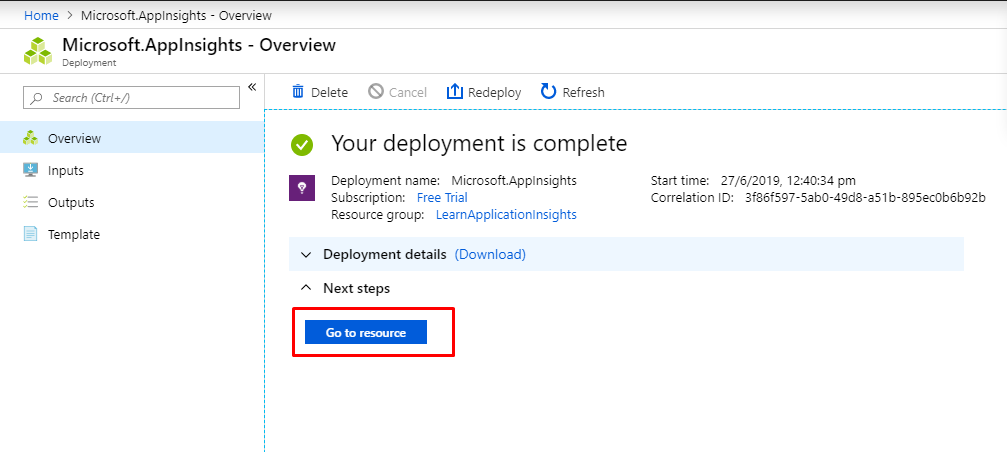
Copy the Instrument Key. We will require this key within our code.
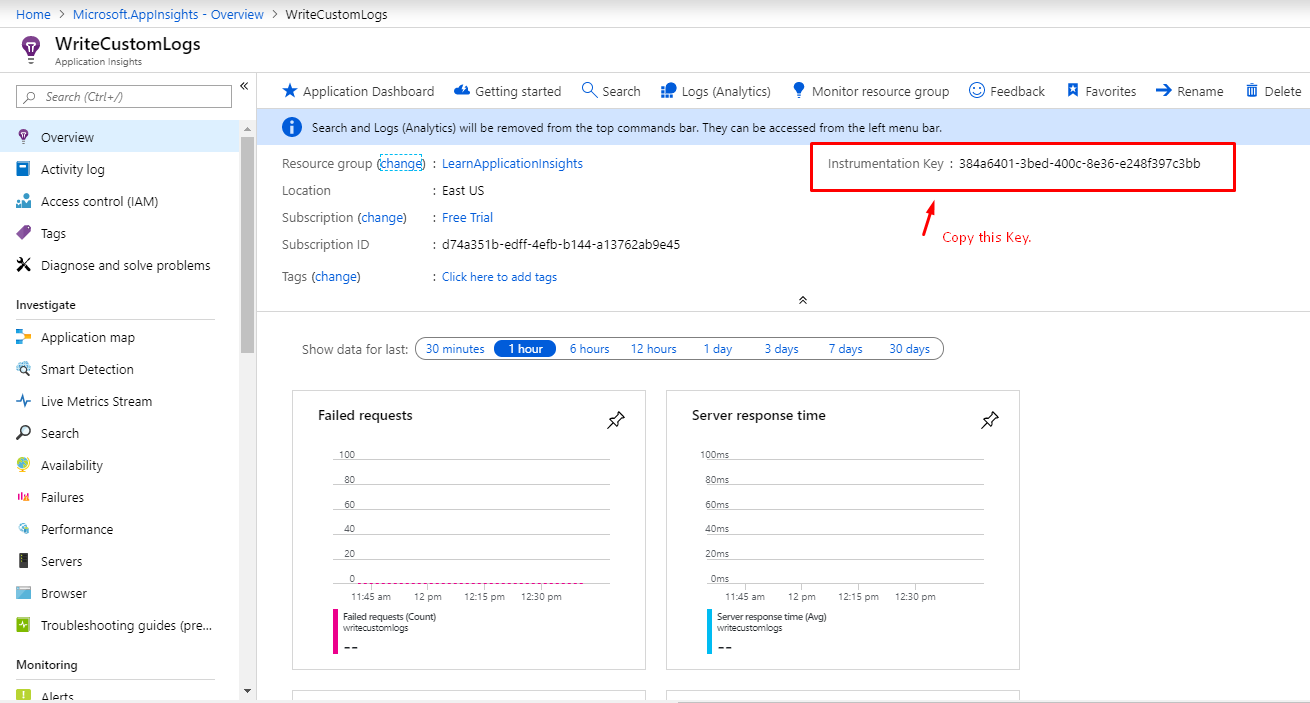
2. Create a Azure Function
Steps :
- Create a new Azure Function
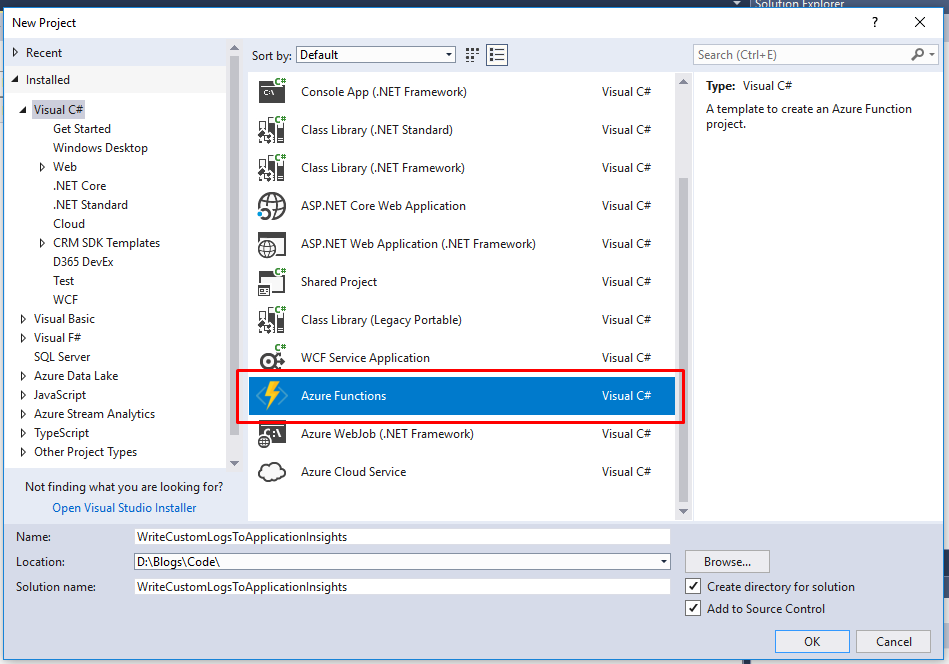
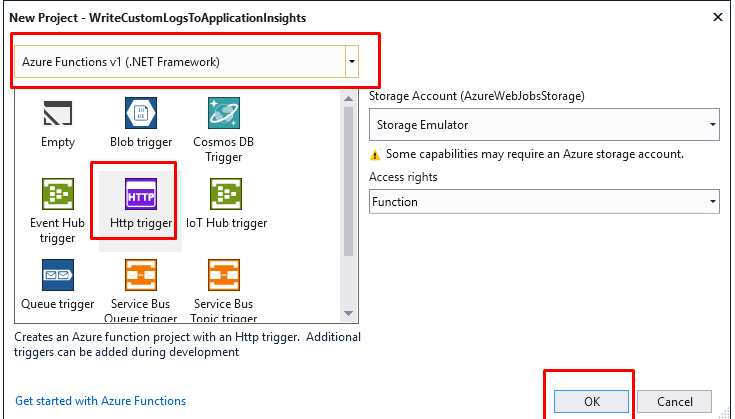
2. Add NuGet Packages Microsoft.ApplicationInsights
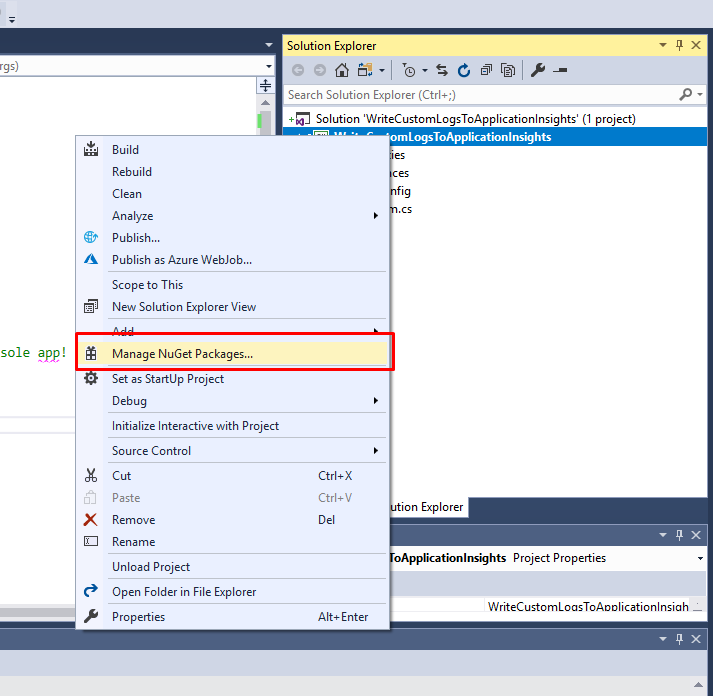
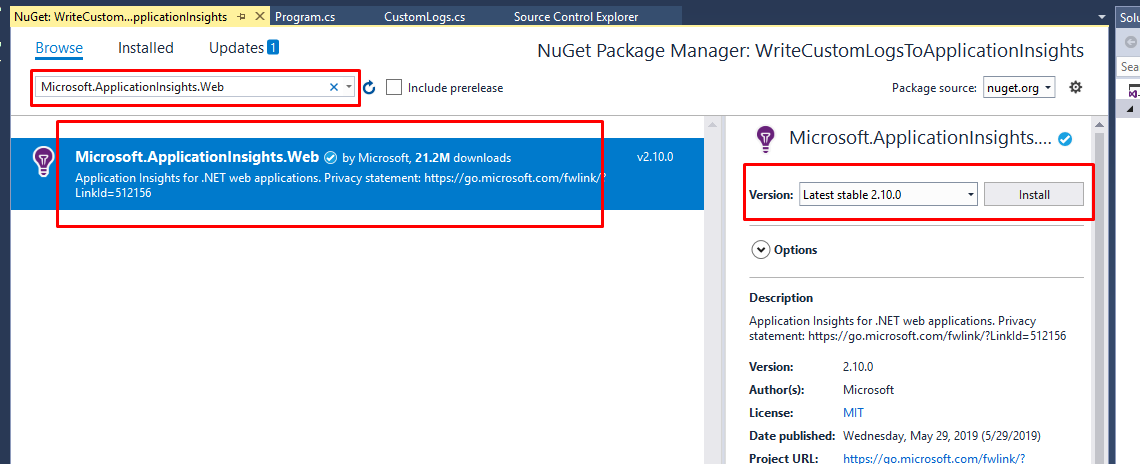

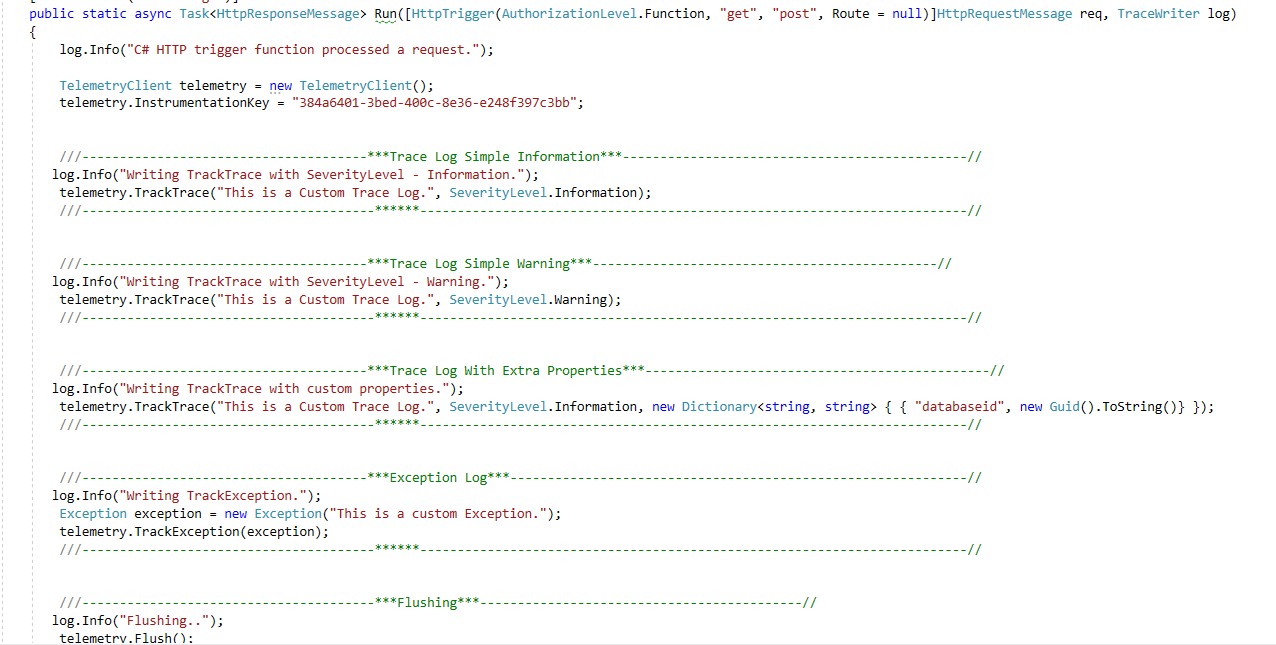
Please download the Code from GitHub
using Microsoft.ApplicationInsights;
using Microsoft.ApplicationInsights.DataContracts;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.Azure.WebJobs.Host;
using System;
using System.Collections.Generic;
using System.Net;
using System.Net.Http;
using System.Threading.Tasks;
namespace WriteCustomLogsToApplicationInsights
{
public static class CustomLogs
{
[FunctionName("CustomLogs")]
public static async Task<HttpResponseMessage> Run([HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)]HttpRequestMessage req, TraceWriter log)
{
log.Info("C# HTTP trigger function processed a request.");
TelemetryClient telemetry = new TelemetryClient();
telemetry.InstrumentationKey = "384a6401-3bed-400c-8e36-e248f397c3bb";
///--------------------------------------***Trace Log Simple Information***----------------------------------------------//
log.Info("Writing TrackTrace with SeverityLevel - Information.");
telemetry.TrackTrace("This is a Custom Trace Log.", SeverityLevel.Information);
///---------------------------------------******-------------------------------------------------------------------------//
///--------------------------------------***Trace Log Simple Warning***----------------------------------------------//
log.Info("Writing TrackTrace with SeverityLevel - Warning.");
telemetry.TrackTrace("This is a Custom Trace Log.", SeverityLevel.Warning);
///---------------------------------------******-------------------------------------------------------------------------//
///--------------------------------------***Trace Log With Extra Properties***----------------------------------------------//
log.Info("Writing TrackTrace with custom properties.");
telemetry.TrackTrace("This is a Custom Trace Log.", SeverityLevel.Information, new Dictionary<string, string> { { "databaseid", new Guid().ToString()} });
///---------------------------------------******-------------------------------------------------------------------------//
///--------------------------------------***Exception Log***-------------------------------------------------------------//
log.Info("Writing TrackException.");
Exception exception = new Exception("This is a custom Exception.");
telemetry.TrackException(exception);
///---------------------------------------******-------------------------------------------------------------------------//
///---------------------------------------***Flushing***-------------------------------------------//
log.Info("Flushing..");
telemetry.Flush();
// Allow some time for flushing before shutdown.
System.Threading.Thread.Sleep(5000);
///---------------------------------------******-------------------------------------------//
log.Info("Completed.");
return req.CreateResponse(HttpStatusCode.OK, "Success ");
}
}
}
3. Provision a Azure Function in Azure Portal and Publish the App from Visual Studio
Steps :
- Login to Azure Portal and Open the Resource Group
- Click on + Add Sign and Search for Azure Functions
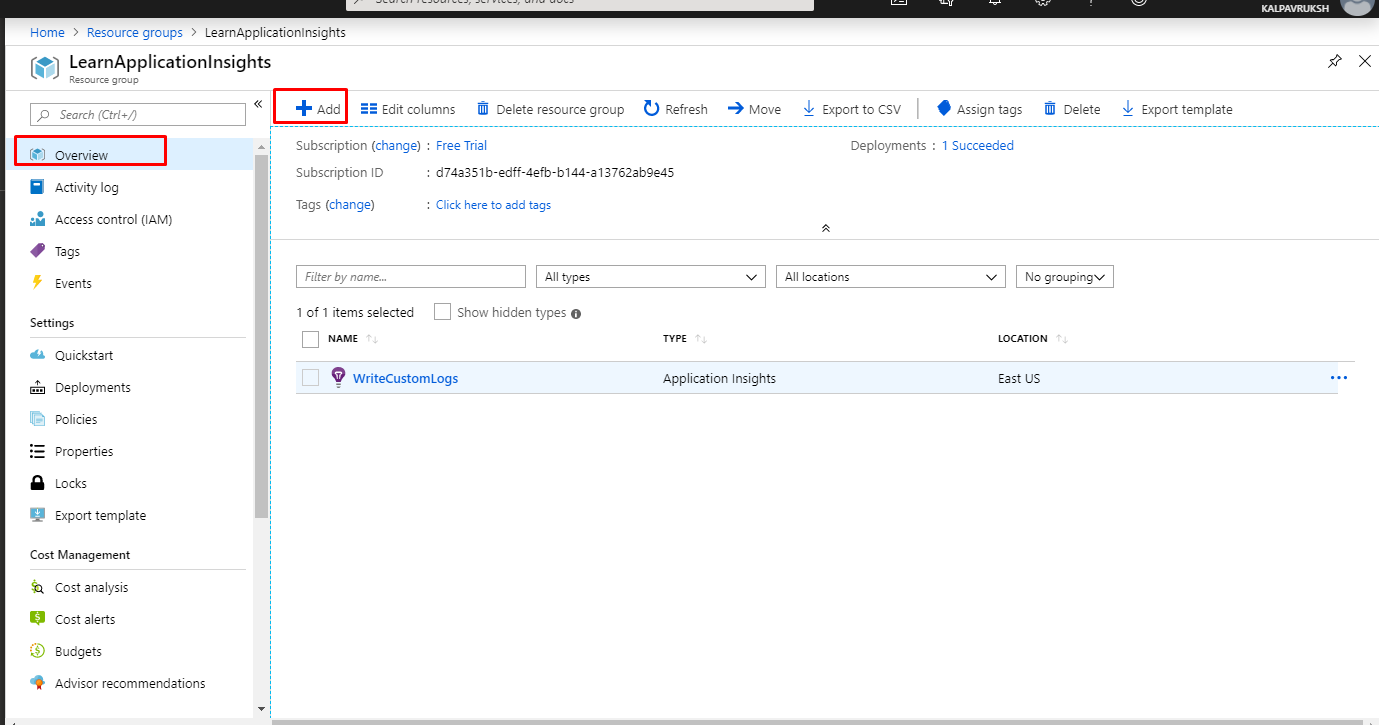
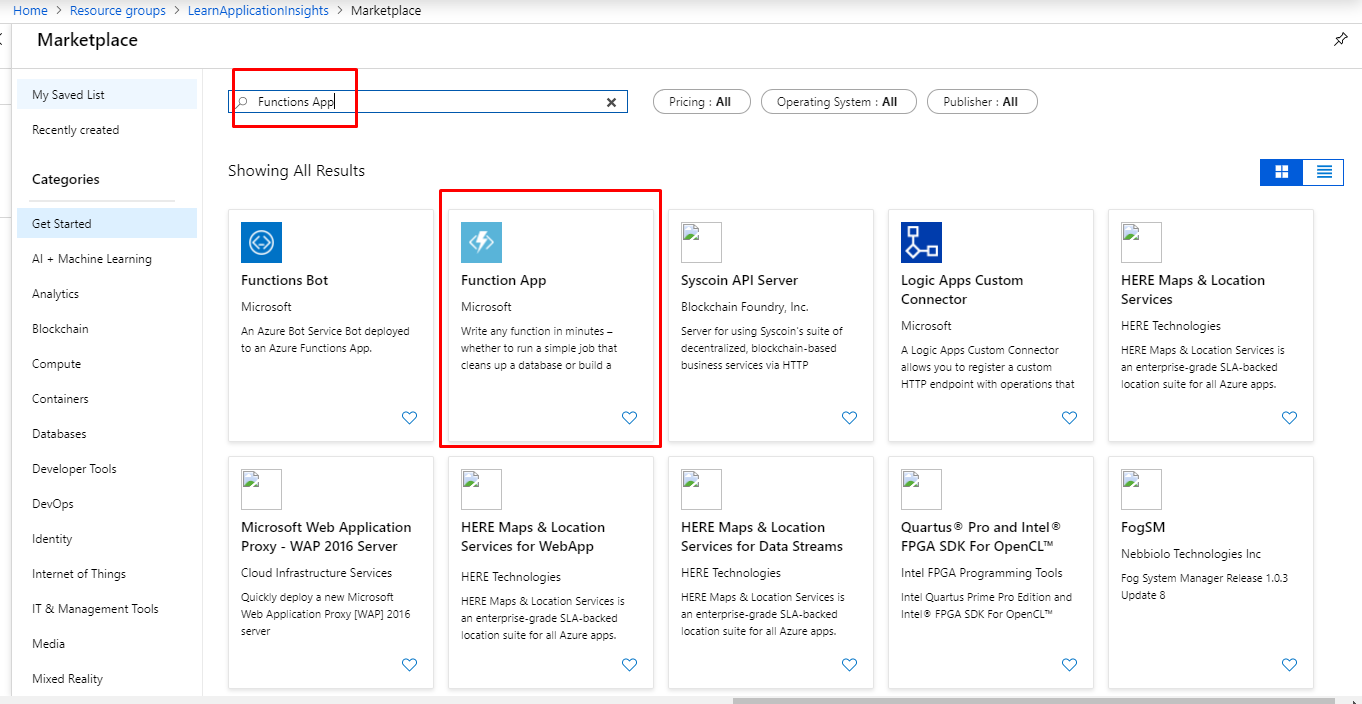
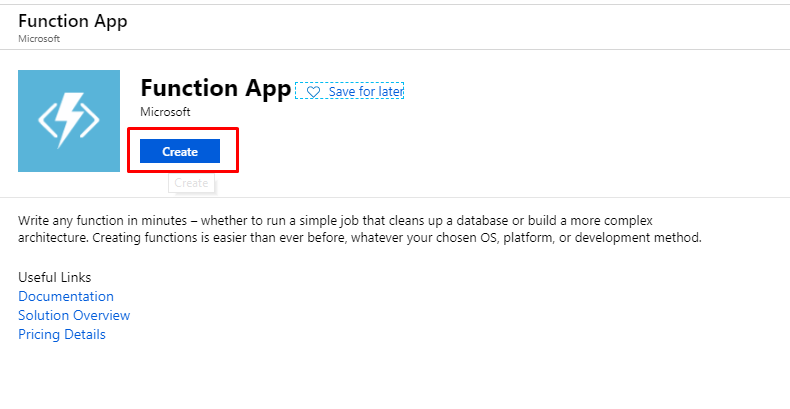
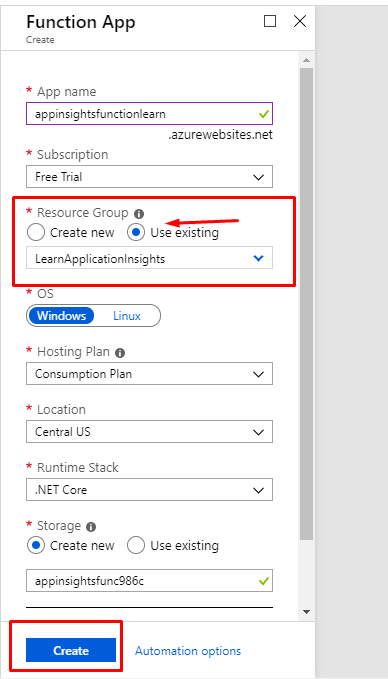
Wait for the Function App to Provision in Azure.
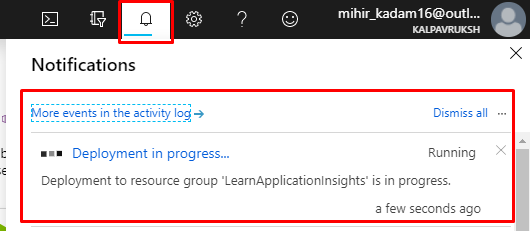
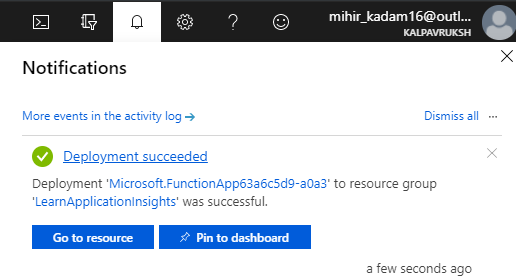
4. Publish the App from Visual Studio
Steps
- Right Click and Publish the App.
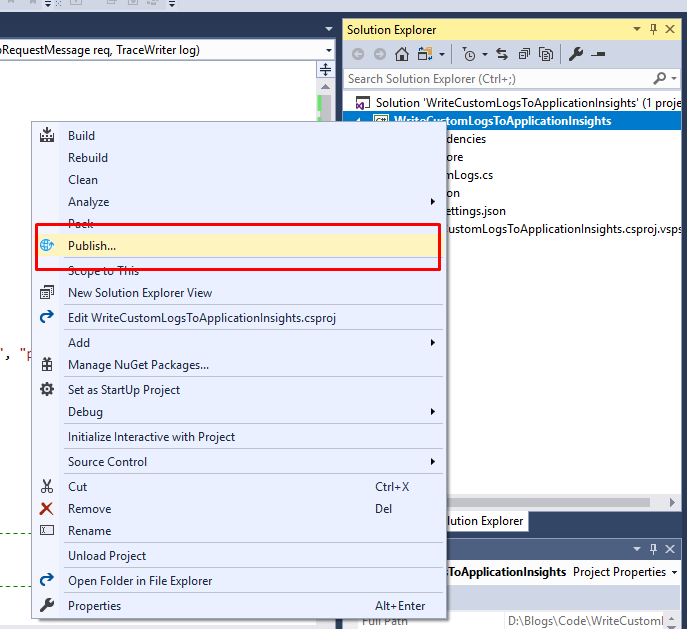
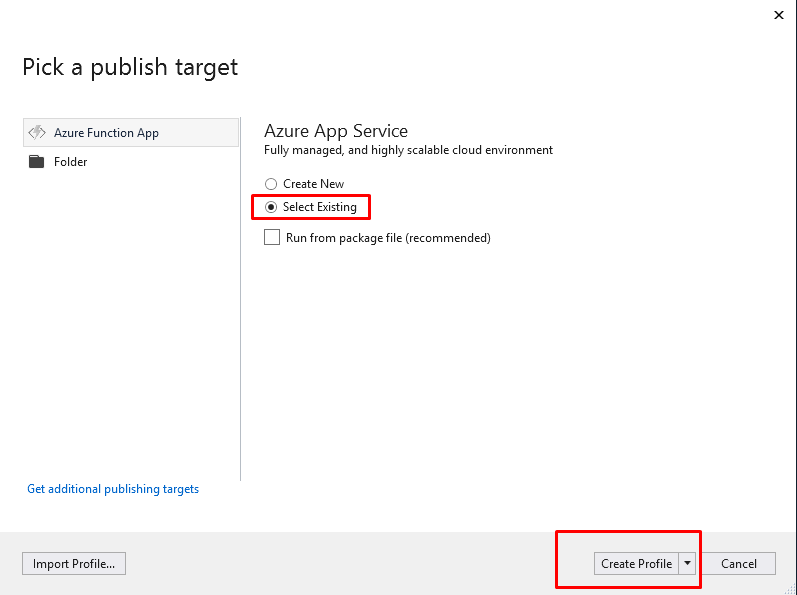
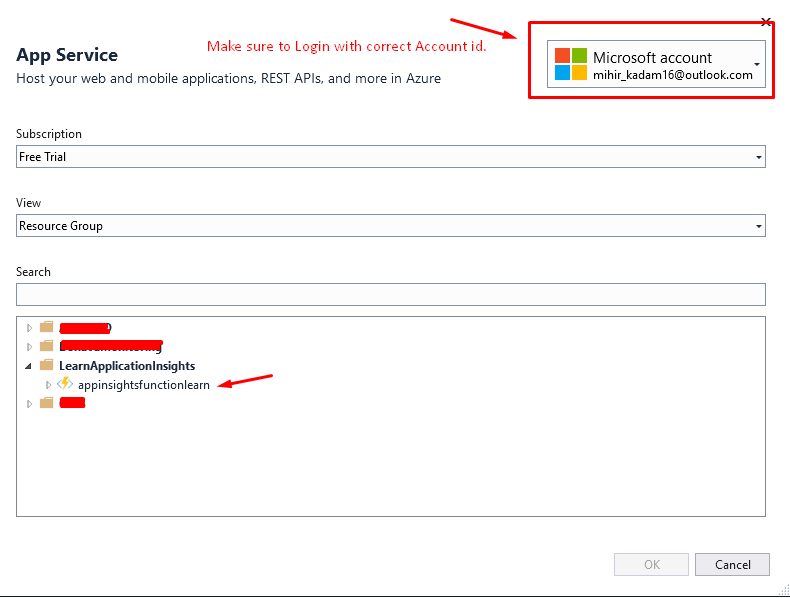
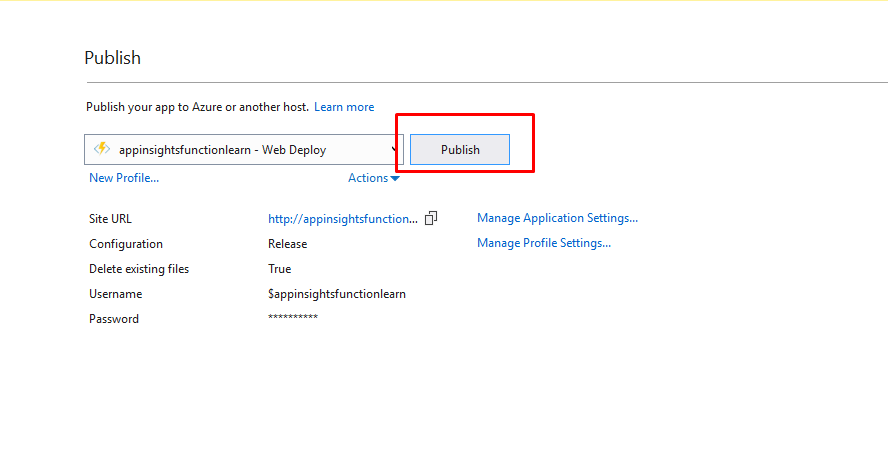
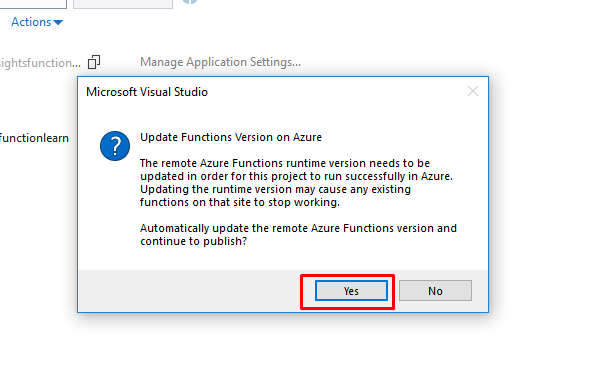
5. Execute the Azure Function from Postman
Steps
- Login to Azure and Open the Azure Function

Get the Function URL
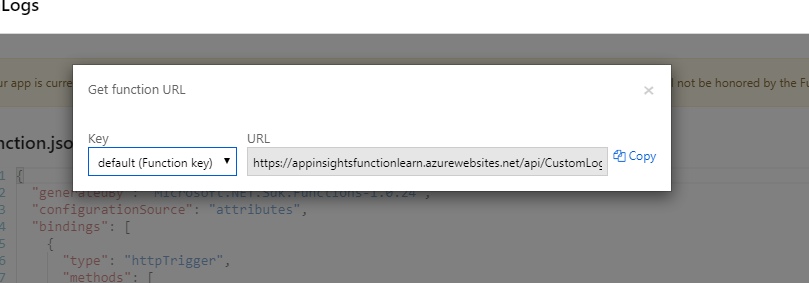
Execute the request from Postman.
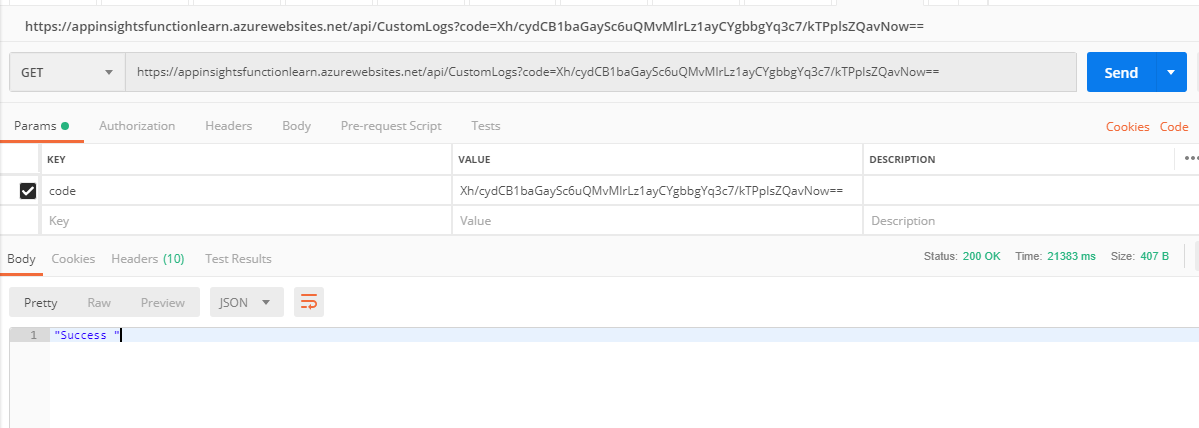
5. Verify the Logs using Logs(Analytics)
Steps
- Open the Application Insights and Click on Logs(Analytics)
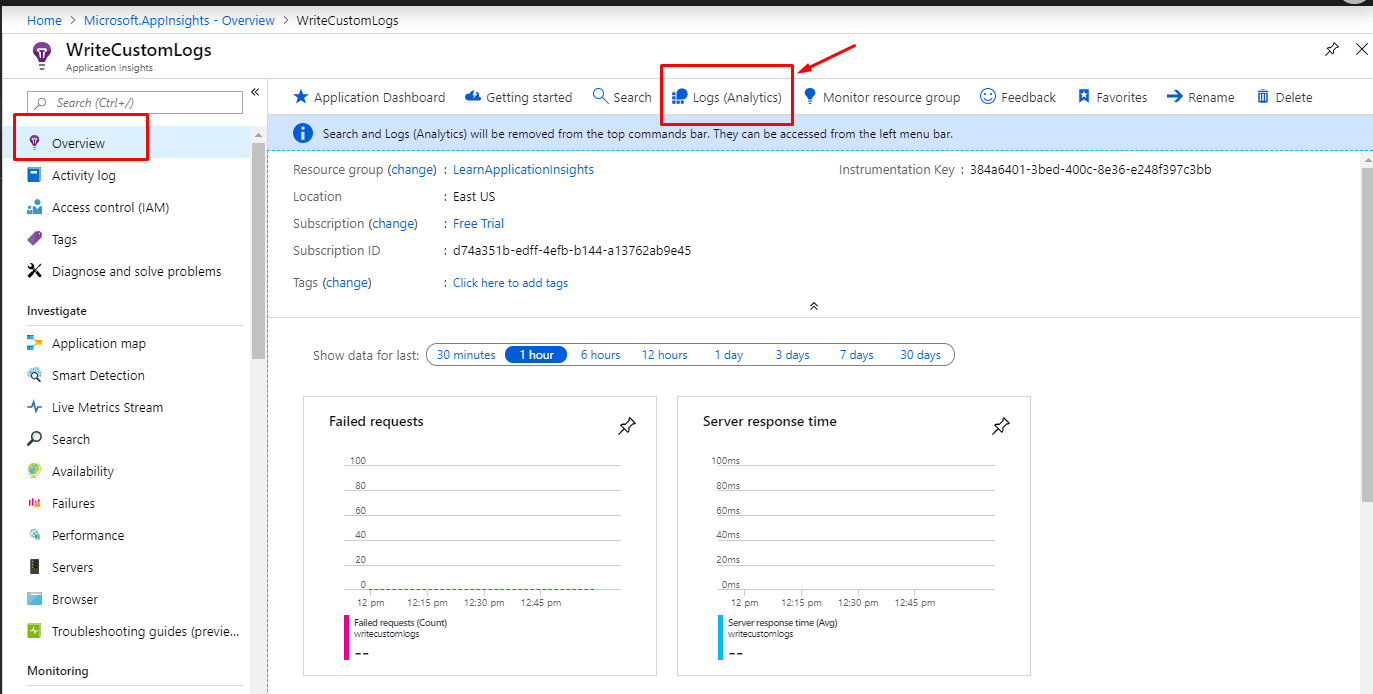
2. Run the Query to verify
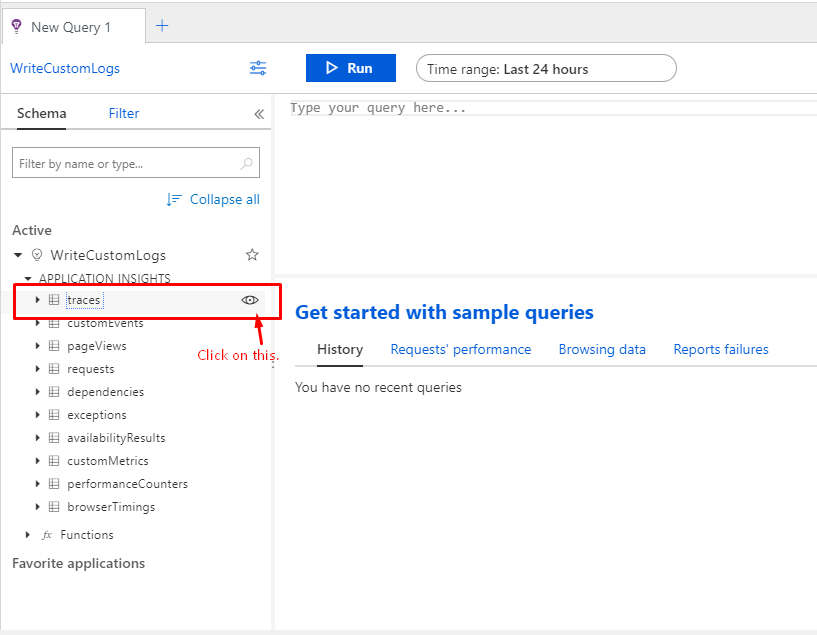
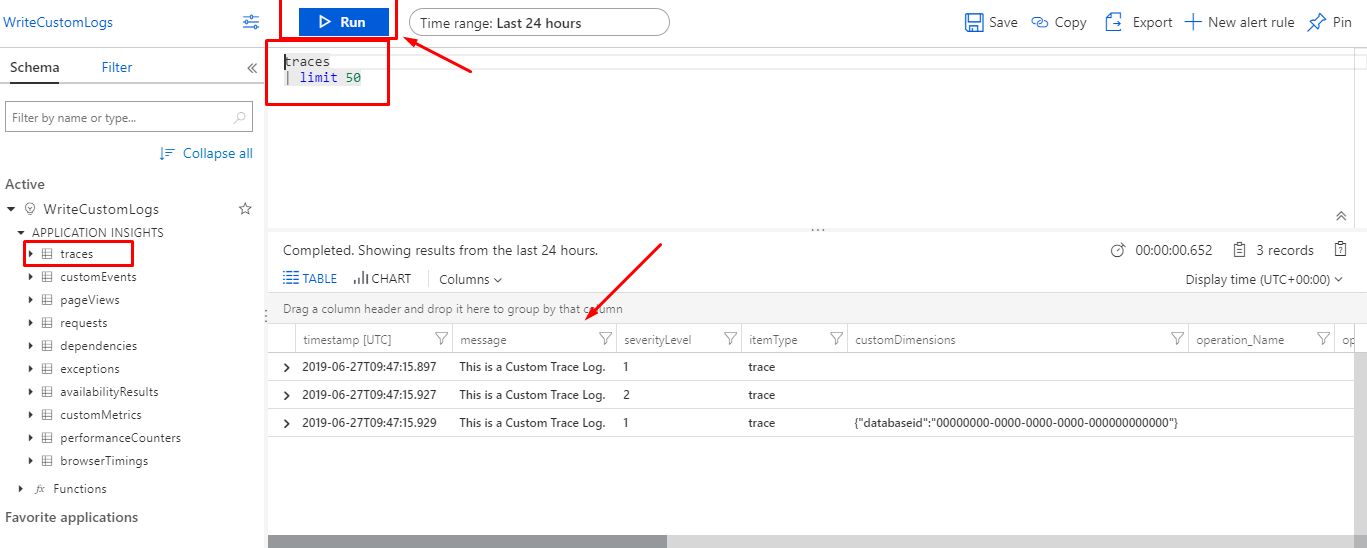
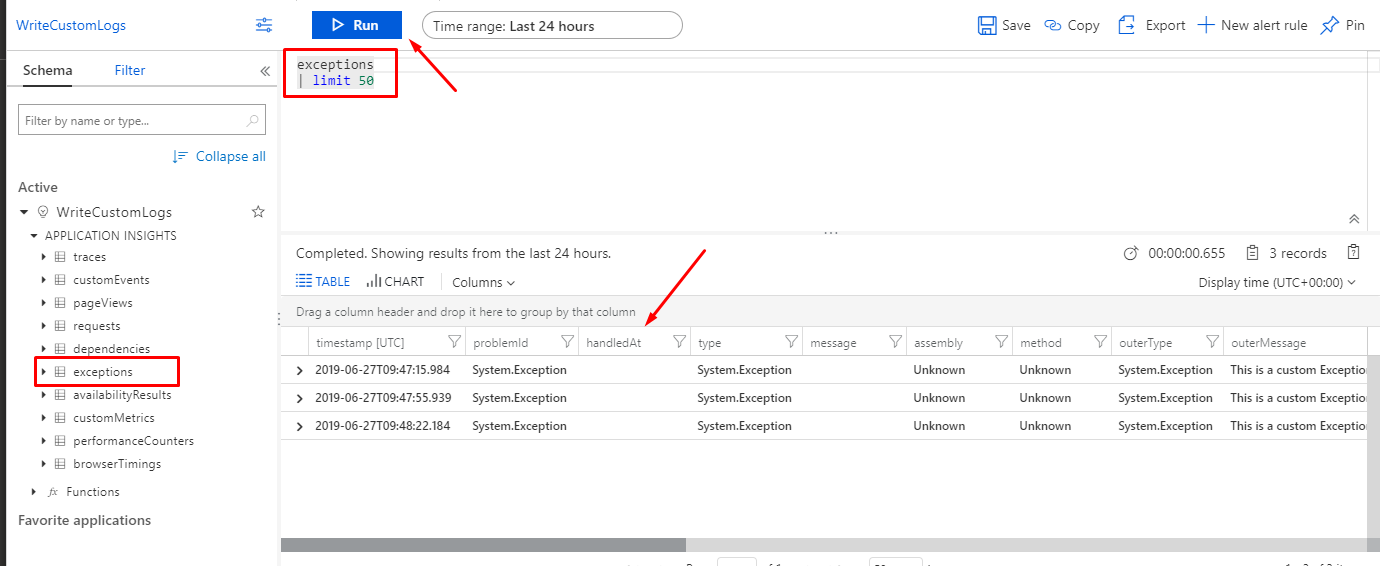
We have successfully captured the custom trace logs and exceptions in Application Insights.
Reference Link
https://docs.microsoft.com/en-us/azure/azure-monitor/app/api-custom-events-metrics#tracktrace
Cheers….. :):)